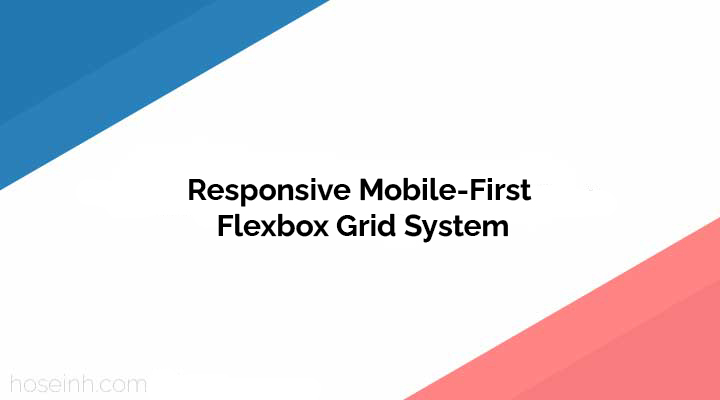
January 5, 2025
Creating a Responsive Mobile-First Flexbox Grid System with SASS
When developing modern web interfaces, it’s crucial to create layouts that are responsive, adaptable to different screen sizes, and optimized for mobile-first design. While frameworks like Bootstrap and TailwindCSS provide convenient, prebuilt grid systems, there are scenarios where you might want to build your own custom grid system without relying on a full UI framework.
Even though CSS Grid has been an extremely useful feature for creating complex layouts, there are scenarios where Flexbox can offer more control, particularly for dynamic content or when dealing with legacy browser support. This post will guide you through creating a flexible, responsive grid using SASS and Flexbox. Furthermore the following solution resembles bootstrap’s grid system which is familiar to many developers.
Why Build a Custom Grid System?
Using a custom grid system allows for more control and flexibility over your layout. You can tailor the grid to your specific needs without the overhead of an entire UI library. By leveraging the power of Flexbox and SASS, you can create a clean, scalable grid system that adapts to all screen sizes with ease.
In this post, we will create a simple Bootstrap-like grid system using SASS that can be easily integrated into your project. We’ll focus on a mobile-first approach, ensuring that the grid is optimized for smaller screens and progressively enhances for larger ones.
The SASS Code for a Custom Flex Grid System
Below is the SASS code that defines a responsive grid system similar to Bootstrap’s grid. It includes breakpoints for different screen sizes and utilizes Flexbox for the layout. You can copy and paste this code into your SASS file:
// Bootstrap-like Flex Grid System
$breakpoint-sm: 576px;
$breakpoint-md: 768px;
$breakpoint-lg: 992px;
$breakpoint-xl: 1200px;
$gutter: 16px;
// Mixin for columns
@mixin make-xy($class) {
@for $i from 1 through 12 {
.#{$class}-#{$i} {
flex: 0 0 calc(#{100% / 12 * $i} - #{$gutter});
max-width: calc(#{100% / 12 * $i} - #{$gutter});
}
}
}
@include make-xy("col");
@media (min-width: $breakpoint-sm) {
@include make-xy("col-sm");
}
@media (min-width: $breakpoint-md) {
@include make-xy("col-md");
}
@media (min-width: $breakpoint-lg) {
@include make-xy("col-lg");
}
@media (min-width: $breakpoint-xl) {
@include make-xy("col-xl");
}
// Row
@mixin make-row() {
.row {
display: flex;
flex-wrap: wrap;
margin-right: -#{$gutter / 2};
margin-left: -#{$gutter / 2};
}
.row > * {
padding-right: #{$gutter / 2};
padding-left: #{$gutter / 2};
}
}
@include make-row();
Explanation of the Code
- Breakpoints: The grid system uses the standard breakpoints:
576px
for small screens,768px
for medium screens,992px
for large screens, and1200px
for extra-large screens. These are adjustable, allowing you to fine-tune the layout for different device sizes. - Columns with Mixin: The
make-xy
mixin generates 12 columns based on a flex system, calculating the width for each column dynamically. This ensures that the grid is responsive and adjusts to different screen sizes. - Responsive Media Queries: Media queries are used to apply the column styles at different breakpoints. For example,
.col-md-4
will make the column take up 4 out of 12 parts of the grid at medium screen sizes. - Row Layout: The
.row
class is styled withflex
to ensure that the columns align properly, and gutter spaces are handled with padding.
Using the Grid System in Your HTML
To implement the grid system in your HTML, simply use the following structure. Here’s an example of how to apply the grid classes to create a responsive layout:
<div class="row">
<div class="col-12 col-md-4 col-lg-2">
1
</div>
<div class="col-12 col-md-4 col-lg-2">
2
</div>
<div class="col-12 col-md-4 col-lg-2">
3
</div>
<div class="col-12 col-md-4 col-lg-2">
4
</div>
</div>
In the example above:
- On small screens (
col-12
), each column takes up the full width. - On medium screens (
col-md-4
), each column takes up 1/3 of the width. - On large screens (
col-lg-2
), each column takes up 1/6 of the width.
This approach provides a responsive layout that adapts to various screen sizes.