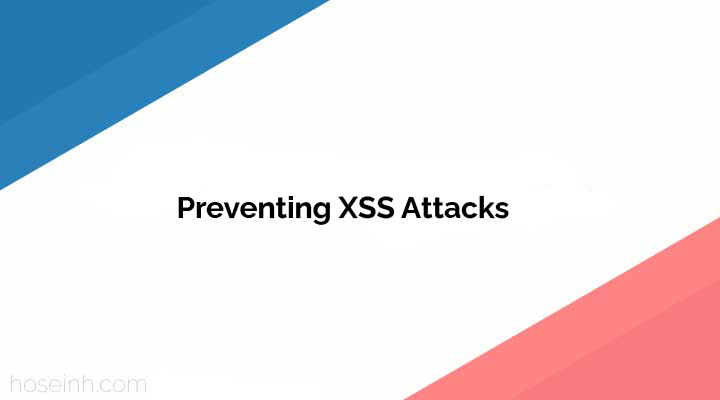
July 3, 2024
Preventing XSS Attacks: Best Practices and Tips
Cross-Site Scripting (XSS) attacks have been a persistent threat to web applications for years, and their prevention is crucial for maintaining the security and integrity of user data. There are various techniques and best practices that developers can implement to mitigate the risk of XSS attacks. In this post, we will discuss the use of HTTP headers, the importance of HttpOnly cookies, input data sanitization, and more.
Utilizing HTTP Headers
HTTP headers are a powerful tool for preventing XSS attacks. Here are some essential headers you should implement:
- Content-Security-Policy (CSP): This header helps you control the resources the user agent is allowed to load for a given page. By specifying trusted sources, you can prevent the execution of malicious scripts. For instance:
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted.cdn.com
- X-Content-Type-Options: This header prevents browsers from MIME-sniffing a response away from the declared content-type. Adding this header ensures that content is interpreted correctly.
X-Content-Type-Options: nosniff
- X-XSS-Protection: Although modern browsers have deprecated this header, it can still be useful for older browsers. It instructs the browser to enable built-in XSS protection.
X-XSS-Protection: 1; mode=block
- Referrer-Policy: This header controls how much referrer information should be included with requests. By limiting this, you can prevent leaking sensitive information.
Referrer-Policy: no-referrer-when-downgrade
HttpOnly Cookies
Cookies are often used to store sensitive information such as session tokens. Marking cookies as HttpOnly ensures that they are inaccessible via JavaScript, thereby reducing the risk of XSS attacks exploiting cookie data.
Set-Cookie: sessionId=abc123; HttpOnly
Sanitizing Input Data
Sanitizing input data is a critical step in preventing XSS attacks. Always treat user input as untrusted and use libraries to sanitize and validate this input. Libraries like DOMPurify for JavaScript can help strip out potentially harmful code from user input.
const cleanInput = DOMPurify.sanitize(userInput);
Avoid Storing Tokens in Local Storage
While local storage is convenient for persisting data, it is accessible through JavaScript, making it vulnerable to XSS attacks. Instead, use secure, HttpOnly cookies for storing tokens and other sensitive information.
Additional Best Practices
- Use Frameworks that Auto-Escape Content: Modern web frameworks like React and Angular automatically escape content, reducing the risk of XSS.
- Content Encoding: Ensure that your server encodes all data before it is sent to the client. This includes HTML, JavaScript, CSS, and JSON.
- Input Validation: Implement robust input validation on both the client and server sides. Ensure that input data conforms to expected formats and ranges.
- Regular Security Audits: Perform regular security audits and code reviews to identify and fix vulnerabilities.
By combining these strategies, you can significantly reduce the risk of XSS attacks and protect your web application from potential threats. Implementing these measures will not only enhance the security of your application but also build trust with your users.