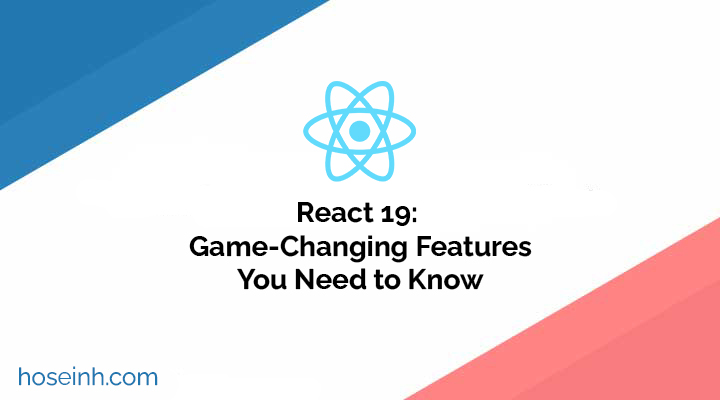
January 26, 2025
React 19: Game-Changing Features You Need to Know
Over two years ago, I reviewed React 18, which introduced significant changes, including new optimization hooks. Now, with the release of React 19 on December 5, 2024, the framework has evolved even further, offering groundbreaking updates designed to make development smoother, faster, and more efficient. From simplified handling of state transitions to new hooks and APIs, this release is packed with features that every React developer should know. In this post, we’ll review the most notable enhancements in this significant update.
1. Actions: Simplifying Async Workflows
One of the common challenges in React apps is managing data mutations alongside state updates. With React 19, the introduction of Actions makes this process significantly easier.
Actions make handling the following easier:
- Pending states: Automatically indicate when a request is in progress.
- Optimistic updates: Provide instant feedback while a request is still in flight.
- Error handling: Display errors and revert changes if a request fails.
- Forms integration: Facilitate form submissions and state resets.
Here’s how Actions and the new useActionState
hook streamline a common use case, such as updating a user’s name:
function ChangeName({ name, setName }) {
const [error, submitAction, isPending] = useActionState(
async (previousState, formData) => {
const error = await updateName(formData.get("name"));
if (error) {
return error;
}
redirect("/profile");
return null;
},
null
);
return (
<form action={submitAction}>
<input type="text" name="name" />
<button type="submit" disabled={isPending}>Update</button>
{error && <p>{error}</p>}
</form>
);
}
This new approach minimizes boilerplate code and allows you to focus on core functionality.
2. useOptimistic Hook: Seamless Optimistic UI Updates
As mentioned earlier actions facilitate handling optimistic updates. The new useOptimistic
hook takes the hassle out of implementing optimistic updates. It lets you render a predicted state while waiting for an asynchronous operation to complete. Once the operation finishes, React automatically reconciles the state.
Example:
function UpdateName({ currentName, onUpdateName }) {
const [optimisticName, setOptimisticName] = useOptimistic(currentName);
const submitAction = async (formData) => {
const newName = formData.get("name");
setOptimisticName(newName);
const updatedName = await updateName(newName);
onUpdateName(updatedName);
};
return (
<form action={submitAction}>
<p>Your name: {optimisticName}</p>
<input type="text" name="name" disabled={currentName !== optimisticName} />
</form>
);
}
This allows for a more responsive UI and better user experience.
3. New <form>
Enhancements
React 19 introduces significant updates to form handling. You can now pass functions directly to the action
or formAction
props, which enables automatic form submission management and state resets.
<form action={submitAction}>
<input type="text" name="name" />
<button type="submit">Submit</button>
</form>
The <form>
element will handle submission, pending states, and errors seamlessly.
4. useFormStatus: A Game-Changer for Design Systems
Speaking of forms, For components in design systems that need access to form statuses, the new useFormStatus
hook provides context-aware insights without prop drilling.
import { useFormStatus } from "react-dom";
import action from './actions';
function Submit() {
const status = useFormStatus();
return <button disabled={status.pending}>Submit</button>
}
export default function App() {
return (
<form action={action}>
<Submit />
</form>
);
}
This feature simplifies building reusable form components. However Note that the useFormStatus Hook currently only provides status information for a parent <form>
and does not support <form>
elements rendered in the same component or in child components.
5. New use
API: Reading Resources in Render
React 19 introduces the use
API for directly reading resources in render. This feature supports promises and context, it makes Suspense even more powerful.
import { use } from 'react';
function Comments({ commentsPromise }) {
const comments = use(commentsPromise);
return comments.map(comment => <p key={comment.id}>{comment}</p>);
}
function Page({ commentsPromise }) {
return (
<Suspense fallback={<div>Loading...</div>}>
<Comments commentsPromise={commentsPromise} />
</Suspense>
);
}
By suspending components until resources are ready, this API ensures smooth and predictable rendering.
6. Server Components and Server Actions
React 19 makes Server Components stable, a groundbreaking feature that allows components to render exclusively on the server before being sent to the client. Unlike traditional client-side components, Server Components run in a separate environment, that enables them to perform operations like data fetching directly within the component. This approach not only reduces the amount of JavaScript sent to the client but also enhances application performance and security by keeping sensitive logic on the server. By integrating Server Components, developers can build applications that seamlessly combine server-rendered and client-rendered components, leading to more efficient and scalable React applications.
Furthermore, Server Actions are a game-changing feature that bridges the gap between client-side components and server-side logic. They allow you to invoke server-side async functions directly from Client Components, which helps reduce client-side bundle sizes and simplify server-client interactions:
"use server";
export async function saveData(data) {
// Server-side logic
await db.save(data);
}
This architecture simplifies server-client communication and reduces client-side bundle sizes. Next.js has already embraced Server components and server actions to make building full-stack react based applications more efficient than ever.
7. Other Notable Enhancements
- Refs as Props: Function components can now accept
ref
as a prop, which makesforwardRef
obsolete in many cases. - Context as a Provider: Use
<Context>
directly as a provider, eliminating the need for<Context.Provider>
. - Document Metadata Support: Metadata tags like
<title>
and<meta>
can now be rendered directly within React components. - Resource Preloading: New APIs like
prefetchDNS
andpreconnect
make it easier to optimize resource loading.
React 19 is a major step forward for the framework and it delivers powerful tools that make development faster and more intuitive. Whether you’re building forms, managing state, or optimizing performance, these features will elevate your React projects to the next level.