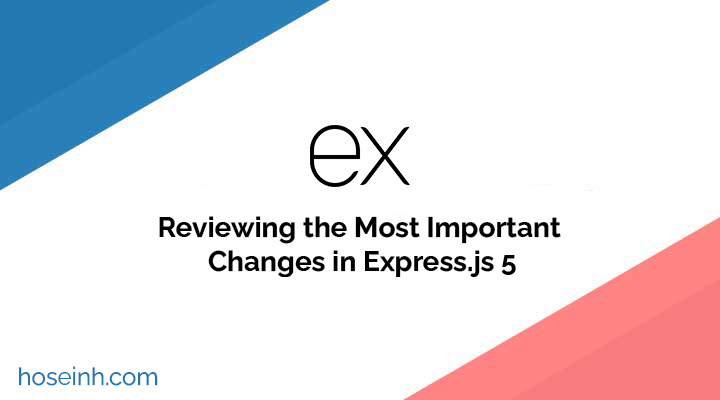
February 8, 2025
Reviewing the Most Important Changes in Express 5
Express.js is the most popular Node.js library for backend development, with millions of installs every month. I’ve been using it for a while now for full-stack development. After over a decade since v4, the new version, Express v5, was released a few months ago with minor improvements and even some breaking changes. However, it remains in the “next” release stage and is not yet considered fully stable.
By default, when you install Express via npm, version 4.21.x is installed. If you want to try Express 5, you need to install it manually:
npm install express@next
In this article, I’ll review the most notable changes and new features in Express 5.
1. Node.js 18 Required
Express 5 has dropped support for older Node.js versions and now requires Node.js 18 or later. This ensures better security, improved performance, and access to the latest ECMAScript features. If you’re still using an older version of Node.js, you’ll need to upgrade before using Express 5.
2. Improved Promise Support
Express 4 already supported promises, but if a promise rejected and returned an error, you had to manually catch it and call the next
function to forward it to your error-handling middleware:
// Route handler
app.get("/posts", async (req, res, next) => {
try {
const data = await loadData();
res.send(data);
} catch (error) {
next(error);
}
});
// Error handling middleware (e.g., errorHandler.js)
app.use((error, req, res, next) => {
res.status(error.status || 500);
res.send({ error: error.message });
});
In Express 5, if a promise fails, the error is automatically forwarded to your global error-handling middleware, eliminating the need for explicit try-catch
blocks:
app.get("/posts", async (req, res) => {
const data = await loadData();
res.send(data);
});
This simplifies code and reduces boilerplate when handling errors in asynchronous route handlers.
3. Removal of Deprecated Method Signatures
Several deprecated method signatures from Express 3 and 4 have been removed in Express 5. You should update your code accordingly:
res.redirect('back')
andres.location('back')
: The magic string'back'
is no longer supported. Usereq.get('Referrer') || '/'
instead.res.send(status, body)
andres.send(body, status)
: Useres.status(status).send(body)
.res.send(status)
: Useres.sendStatus(status)
for simple status responses, orres.status(status).send()
for sending a status code with an optional body.res.redirect(url, status)
: Useres.redirect(status, url)
.res.json(status, obj)
andres.json(obj, status)
: Useres.status(status).json(obj)
.res.jsonp(status, obj)
andres.jsonp(obj, status)
: Useres.status(status).jsonp(obj)
.app.param(fn)
: This method has been deprecated. Instead, access parameters directly viareq.params
, or usereq.body
orreq.query
as needed.app.del('/', () => {})
: Useapp.delete('/', () => {})
instead.
Conclusion
Express 5 brings some important improvements, including native promise error handling, updated method signatures, and compatibility with modern Node.js versions. However, since it’s still in the “next” release stage, it’s best to test it in non-production environments before fully adopting it. If you’re maintaining an Express 4 application, be aware of these changes when planning for future updates.